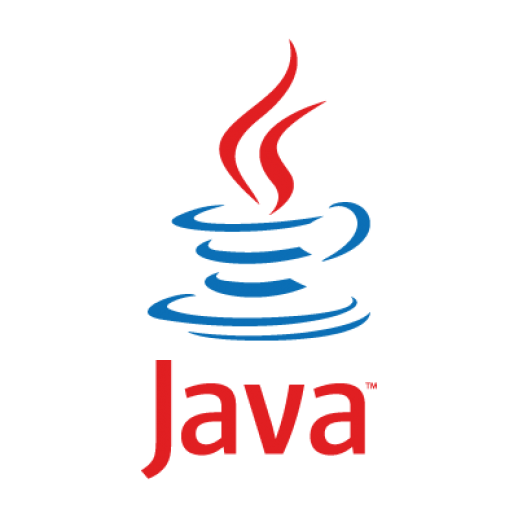
Javaでゲームを作成してみる
公開日:2019年09月18日
更新日:2019年09月24日
手持ちのカードを1枚出して、数字の大きいほうが勝ちというゲーム。
ファイル名:Game.java
import java.util.Collections; import java.util.ArrayList; import java.util.Scanner; import java.util.Random; class Card { private final String MARK; // マーク private final String DISPLAYNUM; // 表示用番号 private int num; // 勝負用の数値 // カード情報初期化 Card(String mark, int num) { this.MARK = mark; this.num = num; switch (num) { case 0: this.DISPLAYNUM = " "; this.num = 99; break; case 1: this.DISPLAYNUM = " A"; this.num = 14; break; case 10: this.DISPLAYNUM = "10"; break; case 11: this.DISPLAYNUM = " J"; break; case 12: this.DISPLAYNUM = " Q"; break; case 13: this.DISPLAYNUM = " K"; break; default: this.DISPLAYNUM = " " + num; break; } } // カードマーク取得 public String getMark() { return this.MARK; } // カード表示用数値取得 public String getDisplayNum() { return this.DISPLAYNUM; } // カード数値取得 public int getNum() { return this.num; } // カード情報表示 public void display() { System.out.println("┌─────────┐"); System.out.println("│ " + this.MARK + " │"); System.out.println("│ " + this.DISPLAYNUM + " │"); System.out.println("└─────────┘"); } } class Player { private String name; // 名前 private int win; // 勝利数 private int lose; // 敗北数 private int draw; // 引き分け数 private ArrayList<Card> cards; // 手持ちのカード private int cardsCount; // 手持ちのカード枚数 // プレイヤーの手札を初期化 Player() { this.name = "コンピューター"; this.win = 0; this.lose = 0; this.draw = 0; this.cards = new ArrayList<Card>(); this.cardsCount = this.cards.size(); } Player(String name) { this(); this.name = name; } // プレイヤーの名前を返す public String getname() { return this.name; } // プレイヤーの勝ち数を返す public int getWin() { return this.win; } // プレイヤーの勝ち数をセットする public void setWin(int num) { this.win = num; } // プレイヤーの負け数を返す public int getLose() { return this.lose; } // プレイヤーの負け数をセットする public void setLose(int num) { this.lose = num; } // プレイヤーの引き分け数を返す public int getDraw() { return this.draw; } // プレイヤーの引き分け数をセットする public void setDraw(int num) { this.draw = num; } // プレイヤーの手札の数を返す public int getCardsCount() { return this.cardsCount; } // プレイヤーの手札を増やす public void addCard(ArrayList<Card> deck) { this.cards.add(deck.get(0)); deck.remove(0); this.cardsCount = this.cards.size(); } // プレイヤーの手札を減らす public void removeCard(int num) { this.cards.remove(num); this.cardsCount = this.cards.size(); } // 選択したプレイヤーの手札を表示 public int displaySingle(int num) { System.out.println("■" + this.name); Card targetCard = this.cards.get(num); targetCard.display(); return targetCard.getNum(); } // プレイヤーの手札をすべて表示 public void displayAll() { System.out.println(); System.out.println("■" + this.name); // カード上部表示 for (int i = 0; i < this.cardsCount + 1; i++) { if (i == 0) { System.out.print("┌─────────"); } else if (i != this.cardsCount) { System.out.print("┬─────────"); } else { System.out.println("┐"); } } // カードマーク表示 for (int i = 0; i < this.cardsCount + 1; i++) { if (i != this.cardsCount) { System.out.print("│ " + this.cards.get(i).getMark() + " "); } else { System.out.println("│"); } } // カード番号表示 for (int i = 0; i < this.cardsCount + 1; i++) { if (i != this.cardsCount) { System.out.print("│ " + this.cards.get(i).getDisplayNum() + " "); } else { System.out.println("│"); } } // カード下部表示 for (int i = 0; i < this.cardsCount + 1; i++) { if (i == 0) { System.out.print("└─────────"); } else if (i != this.cardsCount) { System.out.print("┴─────────"); } else { System.out.println("┘"); } } // カード選択用番号 for (int i = 1; i <= this.cardsCount; i++) { System.out.print(" " + i + " "); } System.out.println(); } } class GameMaster { private final ArrayList<Card> DECK; // トランプカード用変数 private final Player PLAYER; // ゲーム参加者変数:プレイヤー private final Player COMPUTER; // ゲーム参加者変数:コンピューター // 初期化 GameMaster() { Scanner stdIn = new Scanner(System.in); // 入力準備 // トランプカード用リスト:Cardクラスを管理するリスト(配列) this.DECK = new ArrayList<Card>(); // トランプのマーク管理 String[] mark = { " Joker ", " Spade ", " Club ", "Diamond", " Heart ", }; // トランプカード用リストにカードを追加 this.DECK.add(new Card(mark[0], 0)); for (int i = 1; i < mark.length; i++) { for (int j = 1; j <= 13; j++) { this.DECK.add(new Card(mark[i], j)); } } // プレイヤー準備 System.out.println("----------"); System.out.print("名前を入力してください > "); this.PLAYER = new Player(stdIn.next()); this.COMPUTER = new Player(); } // カードシャッフル private void shuffle() { Collections.shuffle(this.DECK); } // カード配布 private void deal(int num) { for (int i = 0; i < num; i++) { this.PLAYER.addCard(this.DECK); this.COMPUTER.addCard(this.DECK); } } // 勝利判定 private String judge(int playerNum, int computerNum) { String message; if (playerNum > computerNum) { this.COMPUTER.setLose(this.COMPUTER.getLose() + 1); this.PLAYER.setWin(this.PLAYER.getWin() + 1); message = "勝利"; } else if (playerNum < computerNum) { this.COMPUTER.setWin(this.COMPUTER.getWin() + 1); this.PLAYER.setLose(this.PLAYER.getLose() + 1); message = "負け"; } else { this.COMPUTER.setDraw(this.COMPUTER.getDraw() + 1); this.PLAYER.setDraw(this.PLAYER.getDraw() + 1); message = "引き分け"; } return message; } // ルール表示 private void displayRule() { System.out.println("----------"); System.out.println("■ルール"); System.out.println("手札から1枚選択し数値の大きい方が勝利"); System.out.println("1枚ジョーカーが入っています"); System.out.println("カードの強さの順番:Joker A K Q J 10 9 8 7 6 5 4 3 2"); } // 総合結果表示 private void displayResult(Player player) { System.out.println("----------"); System.out.println("勝利:" + player.getWin()); System.out.println("敗北:" + player.getLose()); System.out.println("引き分け:" + player.getDraw()); System.out.println("----------"); } // デバッグ用:選択カード表示 private void displaySingle(int num) { System.out.println("■ デバッグ用:選択カード1枚表示"); Card targetCard = this.DECK.get(num); System.out.println(num + ":" + targetCard.getMark() + ":" + targetCard.getDisplayNum()); } // デバッグ用:全カード表示 private void displayAll() { System.out.println("■ デバッグ用:全カード表示"); for (int i = 0; i < this.DECK.size(); i++) { Card targetCard = this.DECK.get(i); System.out.println(i + ":" + targetCard.getMark() + ":" + targetCard.getDisplayNum()); } } // ゲーム開始 public void start() { Random random = new Random(); // 乱数取得準備 Scanner stdIn = new Scanner(System.in); // 入力準備 // カードシャッフル shuffle(); // デバッグ用:全カード表示 // displayAll(); // デバッグ用:1枚カード表示 // displaySingle(0); // ルールの表示 System.out.println("----------"); System.out.print("ルールを確認しますか? 1:する 0:しない > "); if (stdIn.nextInt() == 1) { displayRule(); } // カード配布 System.out.println("----------"); System.out.print("手札何枚でゲームをしますか? > "); deal(stdIn.nextInt()); do { // 勝負の継続 System.out.println("----------"); System.out.print("勝負をしますか? 1:OK 0:NG > "); if (stdIn.nextInt() != 1) { break; } // プレイヤーのカード表示 this.COMPUTER.displayAll(); this.PLAYER.displayAll(); // 勝負カード選択 int computerBet = random.nextInt(this.COMPUTER.getCardsCount()); System.out.println("----------"); System.out.print("何番のカードで勝負しますか? > "); int playerBet = stdIn.nextInt() - 1; // デバッグ用:コンピューター選択番号 System.out.println("----------"); System.out.println("コンピューター選択番号:" + (computerBet + 1)); // 勝負カード表示 System.out.println("----------"); int computerBetCardNum = this.COMPUTER.displaySingle(computerBet); int playerBetCardNum = this.PLAYER.displaySingle(playerBet); // 勝利判定 System.out.println(judge(playerBetCardNum, computerBetCardNum)); // 勝負カード削除 this.COMPUTER.removeCard(computerBet); this.PLAYER.removeCard(playerBet); System.out.println("----------"); System.out.println("残り枚数:" + this.PLAYER.getCardsCount()); } while (this.PLAYER.getCardsCount() > 0); // カードゲーム結果表示 displayResult(this.PLAYER); } } public class Game { public static void main(String[] args) { // ゲーム開始準備 GameMaster round1 = new GameMaster(); // ゲーム開始 round1.start(); } }
コマンドプロンプト実行結果
c:\java\test>java Game ---------- 名前を入力してください > akutsu ---------- ルールを確認しますか? 1:する 0:しない > 1 ---------- ■ルール 手札から1枚選択し数値の大きい方が勝利 1枚ジョーカーが入っています カードの強さの順番:Joker A K Q J 10 9 8 7 6 5 4 3 2 ---------- 手札何枚でゲームをしますか? > 3 ---------- 勝負をしますか? 1:OK 0:NG > 1 ■コンピューター ┌─────────┬─────────┬─────────┐ │ Heart │ Club │ Spade │ │ 7 │ 9 │ 10 │ └─────────┴─────────┴─────────┘ 1 2 3 ■akutsu ┌─────────┬─────────┬─────────┐ │ Spade │ Heart │ Heart │ │ J │ 10 │ J │ └─────────┴─────────┴─────────┘ 1 2 3 ---------- 何番のカードで勝負しますか? > 3 ---------- コンピューター選択番号:2 ---------- ■コンピューター ┌─────────┐ │ Club │ │ 9 │ └─────────┘ ■akutsu ┌─────────┐ │ Heart │ │ J │ └─────────┘ 勝利 ---------- 残り枚数:2 ---------- 勝負をしますか? 1:OK 0:NG > 1 ■コンピューター ┌─────────┬─────────┐ │ Heart │ Spade │ │ 7 │ 10 │ └─────────┴─────────┘ 1 2 ■akutsu ┌─────────┬─────────┐ │ Spade │ Heart │ │ J │ 10 │ └─────────┴─────────┘ 1 2 ---------- 何番のカードで勝負しますか? > 2 ---------- コンピューター選択番号:2 ---------- ■コンピューター ┌─────────┐ │ Spade │ │ 10 │ └─────────┘ ■akutsu ┌─────────┐ │ Heart │ │ 10 │ └─────────┘ 引き分け ---------- 残り枚数:1 ---------- 勝負をしますか? 1:OK 0:NG > 1 ■コンピューター ┌─────────┐ │ Heart │ │ 7 │ └─────────┘ 1 ■akutsu ┌─────────┐ │ Spade │ │ J │ └─────────┘ 1 ---------- 何番のカードで勝負しますか? > 1 ---------- コンピューター選択番号:1 ---------- ■コンピューター ┌─────────┐ │ Heart │ │ 7 │ └─────────┘ ■akutsu ┌─────────┐ │ Spade │ │ J │ └─────────┘ 勝利 ---------- 残り枚数:0 ---------- 勝利:2 敗北:0 引き分け:1 ----------
同じカテゴリーのコンテンツ