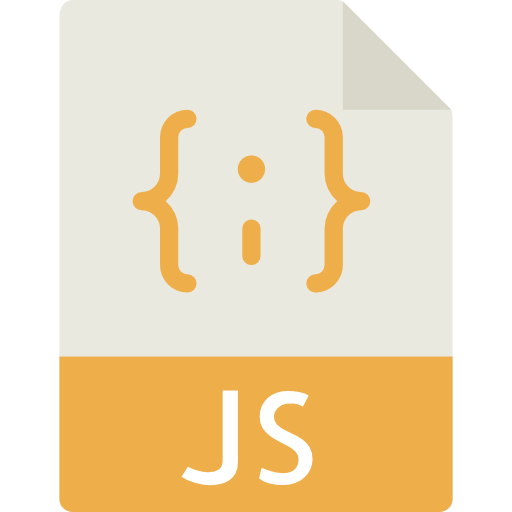
スムーススクロールを作る
準備
jQueryで作成するので「jQuery」本体を読み込む
</body>の直前(推奨)
jquery.min.js:jQuery 本体
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
jquery.easing.min.js:移動時の動きを追加するためのファイルの読み込み
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-easing/1.4.1/jquery.easing.min.js"></script>
smoothscroll.js:今回作成するファイルの読み込み
<script src="js/smoothscroll.js"></script>
コード作成
「smoothscroll.js」内にスムーススクロール用のコードを作成
$(function () {
$('a[href^="#"]').on('click', function () {
let headerHeight = 0; // 固定ヘッダの高さ
let speed = 1000; // 移動スピード
let easing = 'swing'; // 移動時の動き
let href = $(this).attr('href');
let target = $((href == '#' || href == '#top') ? 'html' : href);
let position = target.offset().top;
$('body,html').stop().animate({ scrollTop: position - headerHeight }, speed, easing);
return false;
});
});
固定ヘッダの高さ
固定ヘッダを使用時、変数headerHeightに固定ヘッダーの高さを代入して移動位置のズレを調整します。
$(function () {
$('a[href^="#"]').on('click', function () {
let headerHeight = 0; // 固定ヘッダの高さ
let speed = 1000; // 移動スピード
let easing = 'swing'; // 移動時の動き
let href = $(this).attr('href');
let target = $((href == '#' || href == '#top') ? 'html' : href);
let position = target.offset().top;
$('body,html').stop().animate({ scrollTop: position - headerHeight }, speed, easing);
return false;
});
});
移動スピード
変数speedに移動開始から終了までのスピードをミリ秒で指定して代入します。
$(function () {
$('a[href^="#"]').on('click', function () {
let headerHeight = 0; // 固定ヘッダの高さ
let speed = 1000; // 移動スピード
let easing = 'swing'; // 移動時の動き
let href = $(this).attr('href');
let target = $((href == '#' || href == '#top') ? 'html' : href);
let position = target.offset().top;
$('body,html').stop().animate({ scrollTop: position - headerHeight }, speed, easing);
return false;
});
});
移動時の動き(easing)の設定
変数easingに動きのキーワードを代入して設定します。
$(function () {
$('a[href^="#"]').on('click', function () {
let headerHeight = 0; // 固定ヘッダの高さ
let speed = 1000; // 移動スピード
let easing = 'swing'; // 移動時の動き
let href = $(this).attr('href');
let target = $((href == '#' || href == '#top') ? 'html' : href);
let position = target.offset().top;
$('body,html').stop().animate({ scrollTop: position - headerHeight }, speed, easing);
return false;
});
});
easing用キーワード
基本
linear, swing, jswing
追加:「jquery.easing.min.js」読み込みで使用可能
動きを紹介しているサイト: https://ozpa-h4.com/demo/easing/
easeInQuad, easeOutQuad, easeInOutQuad, easeInCubic, easeOutCubic, easeInOutCubic,easeInQuart, easeOutQuart, easeInOutQuart, easeInQuint, easeOutQuint, easeInOutQuint,easeInSine, easeOutSine, easeInOutSine, easeInExpo, easeOutExpo, easeInOutExpo,easeInCirc, easeOutCirc, easeInOutCirc, easeInElastic, easeOutElastic, easeInOutElastic,easeInBack, easeOutBack, easeInOutBack, easeInBounce, easeOutBounce, easeInOutBounce,